Nokia 5110 LCD is a cheap and simple to use component that you can use in almost all your Arduino projects. There are lots of examples about it, but most of them are a bit complicated because it's used with other components. All I need is a simple 'Hello World' application to start with. Then I can build my complicated applications later. So let's start with Hello World.
What we need?
- Arduino Nano
- Nokia 5110 LCD
- u8glib library. Download: https://bintray.com/olikraus/u8glib/Arduino
After downloading the library, follow Sketch>Include Library>Add .zip Library
menu to include the library. I've also written how to use page loop in u8glib to write more sophisticated applications:
http://cuneyt.aliustaoglu.biz/en/how-to-manage-picture-loop-using-u8glib/
But this article will focus on using Nokia 5110 rather than u8glib.
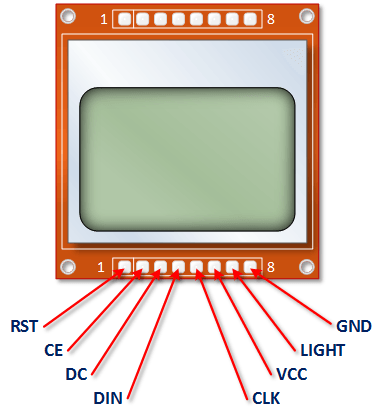
Nokia 5110 | Colour | Arduino Nano |
---|---|---|
RST | Yellow | D7 |
CE | Green | D8 |
DC | Blue | D9 |
Din | White | D10 |
Clk | Purple | D11 |
VCC | Red | 3V3 |
BL | Brown | 3V3 |
Gnd | Black | GND |
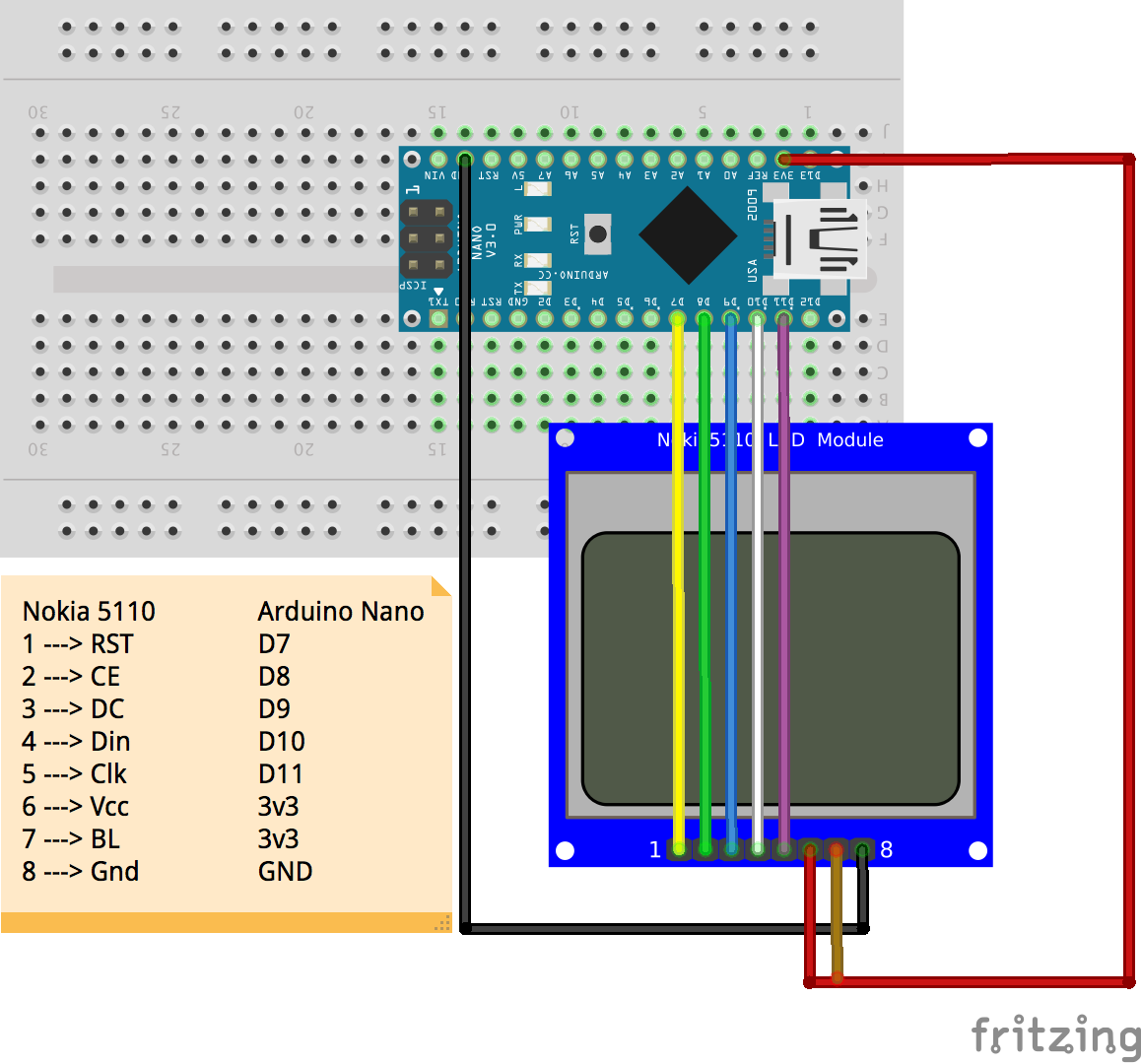
And below is a Nokia 5110 LCD example with u8glib in its simplest form.
#include "U8glib.h"
U8GLIB_PCD8544 u8g(11, 10, 8, 9, 7); // Clk, Din, DC, CE, RST
void draw()
{
u8g.setFont(u8g_font_unifont);
u8g.drawStr(0, 20, "Merhaba ");
u8g.drawStr(12, 36, "Dunya");
}
void setup()
{
u8g.setColorIndex(1);
u8g.setRot180(); // Ekranı 180 derece çevir
}
void loop()
{
u8g.firstPage();
do
{
draw();
}
while(u8g.nextPage());
delay(1000);
}
Now we're ready to add some sensors and output their values into Nokia 5110 LCD.