This tutorial focuses on how to use 1.44'' colour TFT screen 128x128, also known as Nokia 5110 replacement, using JavaScript (Espruino firmware). It's quite a cheap module around 3-5 $. You can easily replace it with Nokia 5110 in your existing projects by keeping the connections exactly the same. With little code change you can get cool, colourful and graphical outputs.
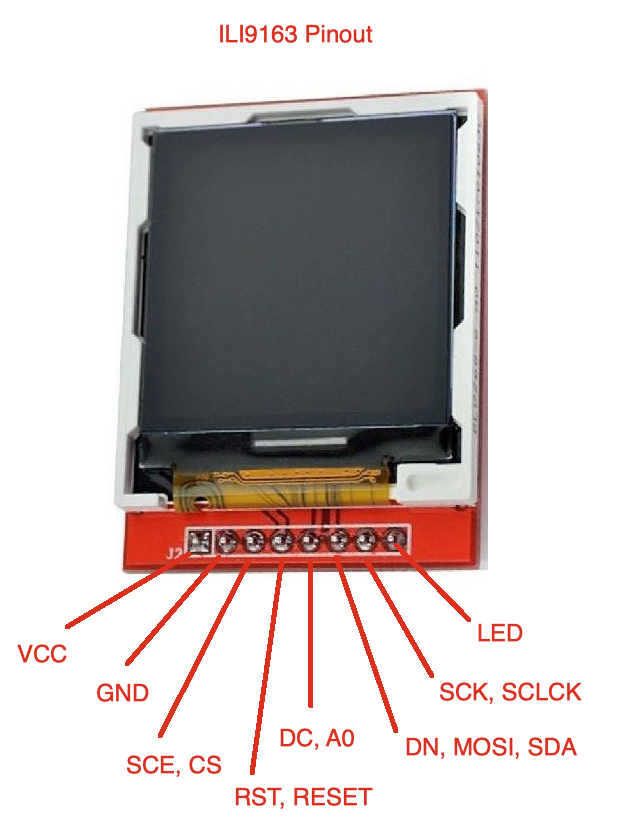
As the firmware, I am using JavaScript. This firmware is called Espruino. In a previous tutorial I have explained how to flash and use this firmware. You can read this tutorial first if you cannot figure out how to flash this firmware.
https://cuneyt.aliustaoglu.biz/en/programming-esp8266-using-javascript-with-espruino/
I made the connections as below. I am using bare ESP-12e module but it if you want to use it with NodeMCU I have noted the corresponding pins.
Nokia-5110 | NodeMCU | ESP-12 | Nokia-Replacement (ILI9163) |
---|---|---|---|
LED | 3V3 | 3V3 | LED |
SCLCK | D3 | GPIO00 | SCK |
DN |
D4 | GPIO02 | SDA |
D/C | D2 | GPIO04 | A0 |
RST | D1 | GPIO05 | RST |
SCE | D8 | GPIO15 | CS |
GND | GND | GND | GND |
VCC | 3V3 | 3V3 | VCC |
And here is the code that prints 3 keywords of this tutorial in different colours:
E.on('init', function() {
var spi = new SPI();
spi.setup({ sck: NodeMCU.D3, mosi: NodeMCU.D4, baud: 115200 });
var g = require('ILI9163').connect(
spi,
NodeMCU.D2 /* RS / DC */,
NodeMCU.D8 /* CS / CE */,
NodeMCU.D1 /*RST*/,
function() {
g.clear();
g.setRotation(90);
g.setFontVector(18);
g.setColor(1, 0, 0);
g.drawString('ESP8266', 0, 10);
g.setColor(0, 1, 0);
g.drawString('Javascript', 0, 40);
g.setColor(0, 0, 1);
g.drawString('TFT', 0, 60);
}
);
digitalWrite(2, 0);
});
You can find this repository below. I automating my tasks with uglify and npm and you might find it useful too.