If you use react-native-maps in React Native, by default you'll be using Apple Maps for IOS and Google Maps for Android. If you want to use Google Maps for IOS, you'll need to make some changes. Like most things in XCode, this is can get a bit tricky.
This tutorial assumes that you're already using Apple Maps in your IOS part of React Native project. You want to use provider as google.
<MapView
mapType="satellite"
provider="google"
style={StyleSheet.absoluteFillObject}
...
/>
When you make this change, your beautiful map will not render anymore and you'll get below screen:
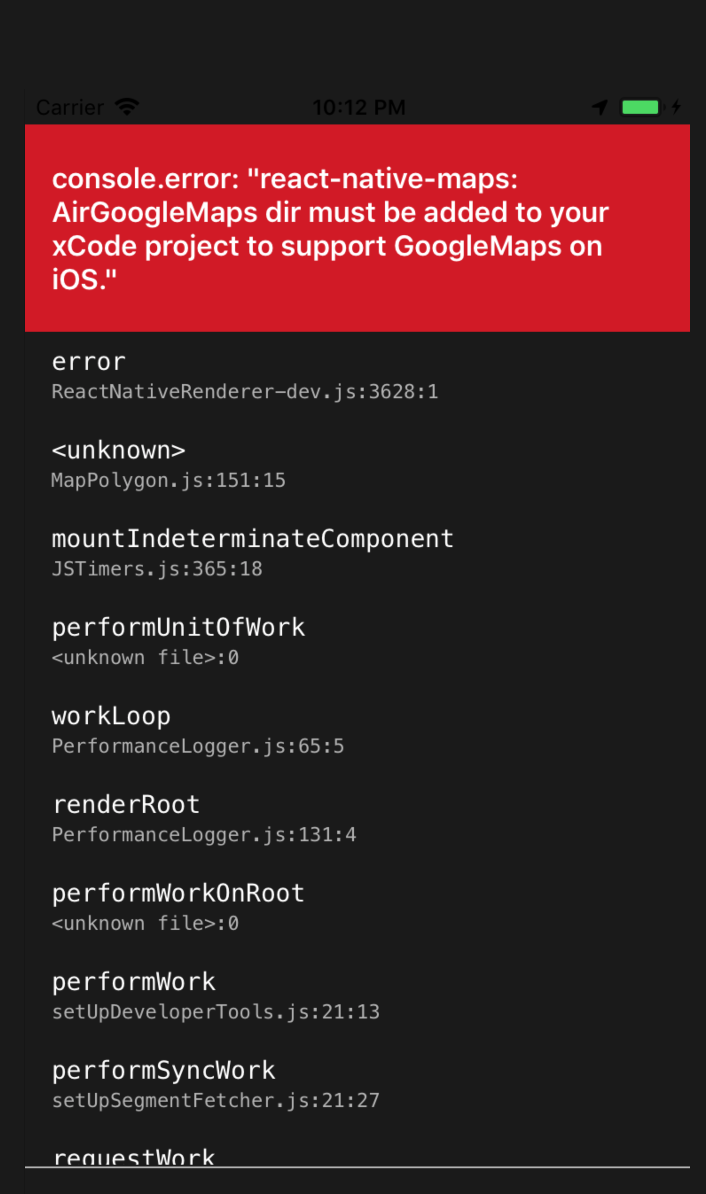
console.error: "react-native-maps: AirGoogleMaps dir must be added to your XCode project to support GoogleMaps on iOS"
First of all add a line `pod 'GoogleMaps'` to your Podfile. Below is my whole podfile:
# File contents of "ios/Podfile"
platform :ios, '9.0'
target 'worldsurfforecast' do
pod 'React', :path => '../node_modules/react-native', :subspecs => [
'Core',
'CxxBridge',
'DevSupport',
# the following ones are the ones taken from "Libraries" in Xcode:
'RCTAnimation',
'RCTActionSheet',
'RCTBlob',
'RCTGeolocation',
'RCTImage',
'RCTLinkingIOS',
'RCTNetwork',
'RCTSettings',
'RCTText',
'RCTVibration',
'RCTWebSocket'
]
pod 'GoogleMaps' #ADD THIS LINE TO YOUR CODE IF YOU WANT GOOGLEMAPS IN IOS
# the following dependencies are dependencies of React native itself.
pod 'yoga', :path => '../node_modules/react-native/ReactCommon/yoga/Yoga.podspec'
pod 'DoubleConversion', :podspec => '../node_modules/react-native/third-party-podspecs/DoubleConversion.podspec'
pod 'Folly', :podspec => '../node_modules/react-native/third-party-podspecs/Folly.podspec'
pod 'glog', :podspec => '../node_modules/react-native/third-party-podspecs/GLog.podspec'
# your other libraries will follow here!
end
# The following is needed to ensure the "archive" step works in XCode.
# It removes React from the Pods project, as it is already included in the main project.
post_install do |installer|
installer.pods_project.targets.each do |target|
if target.name == "React"
target.remove_from_project
end
end
end
To be sure that pods are installed correctly run below commands:
cd ios
rm -rf Pods/
pod install
Now how to add the folder to XCode?
Navigate to node_modules/react-native-maps/lib/ios
and find the folder AirGoogleMaps
. Move the folder under your <ProjectName>. Choose the option "Create Groups" when moving:
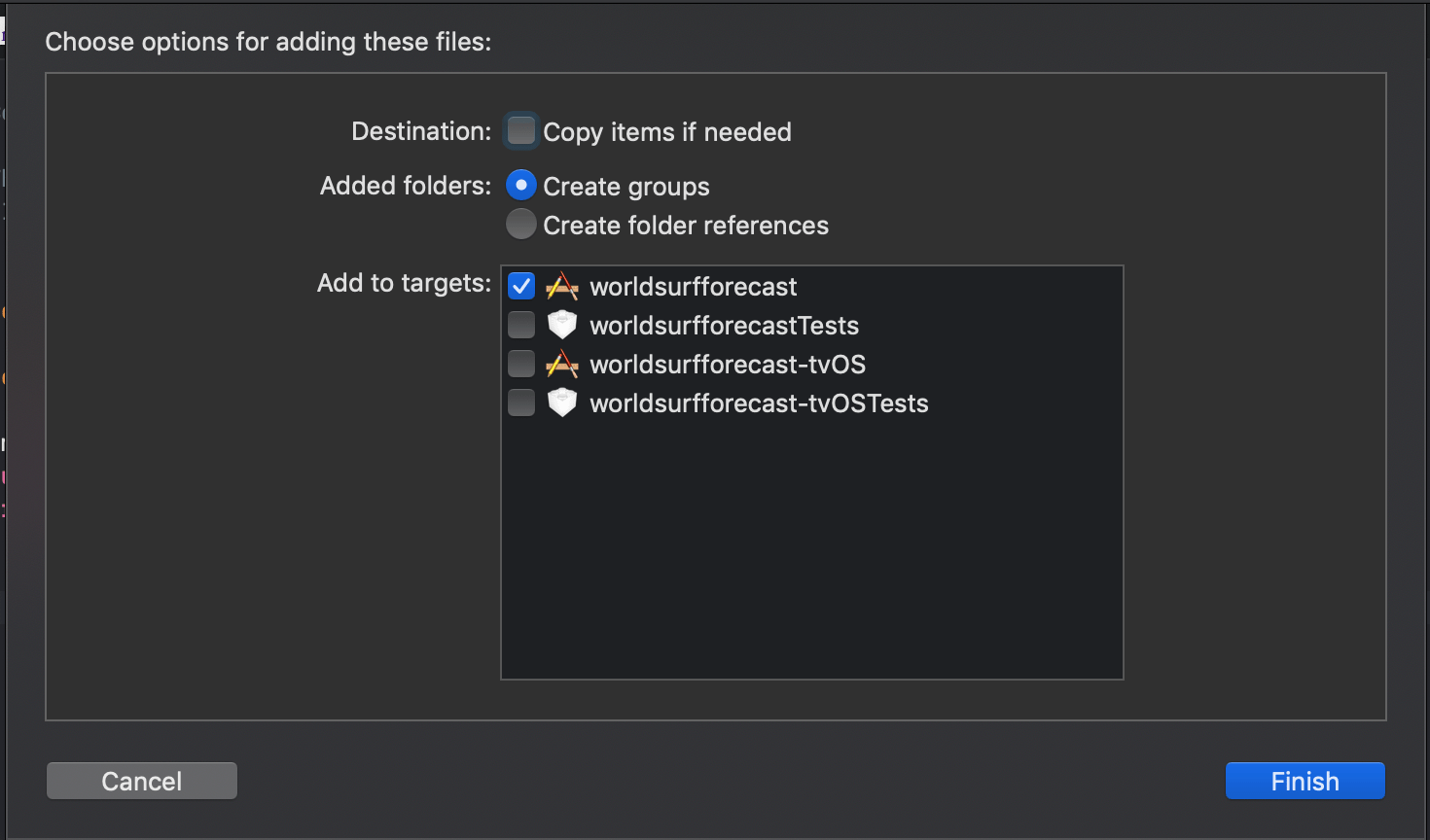
So you'll see "AirGoogleMaps" folder with the same colour as the rest of the folders.
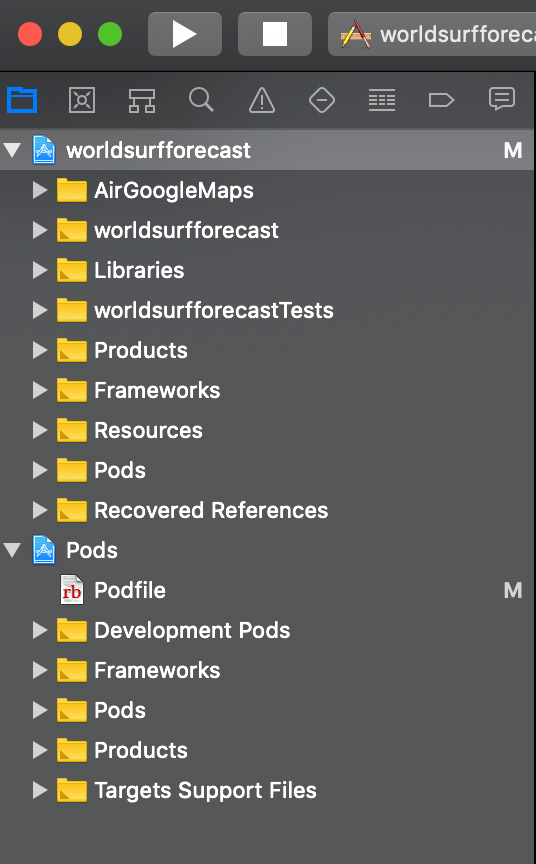
Also we need to provide API key to the native code for IOS. Find file AppDelegate.m
and make below changes:
Add line @import GoogleMaps;
before @implementation AppDelegate
Add line [GMSServices provideAPIKey:@"YOUR_API_KEY"];
after NSURL *jsCodeLocation;
So, it will look like below:
/**
* Copyright (c) Facebook, Inc. and its affiliates.
*
* This source code is licensed under the MIT license found in the
* LICENSE file in the root directory of this source tree.
*/
#import "AppDelegate.h"
#import <React/RCTBundleURLProvider.h>
#import <React/RCTRootView.h>
@import GoogleMaps;
@implementation AppDelegate
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions
{
NSURL *jsCodeLocation;
[GMSServices provideAPIKey:@"AIzaSyDbTO1Zu-zBBmX9hgk-uUpZz001i-V3Ffo"];
jsCodeLocation = [[RCTBundleURLProvider sharedSettings] jsBundleURLForBundleRoot:@"index" fallbackResource:nil];
RCTRootView *rootView = [[RCTRootView alloc] initWithBundleURL:jsCodeLocation
moduleName:@"worldsurfforecast"
initialProperties:nil
launchOptions:launchOptions];
rootView.backgroundColor = [UIColor blackColor];
self.window = [[UIWindow alloc] initWithFrame:[UIScreen mainScreen].bounds];
UIViewController *rootViewController = [UIViewController new];
rootViewController.view = rootView;
self.window.rootViewController = rootViewController;
[self.window makeKeyAndVisible];
return YES;
}
@end
Finally add HAVE_GOOGLE_MAPS=1
to Preprocessor Macros both for debug and release. That is found under Build Settings > Preprocessor Macros. Make sure All selected not Basic.
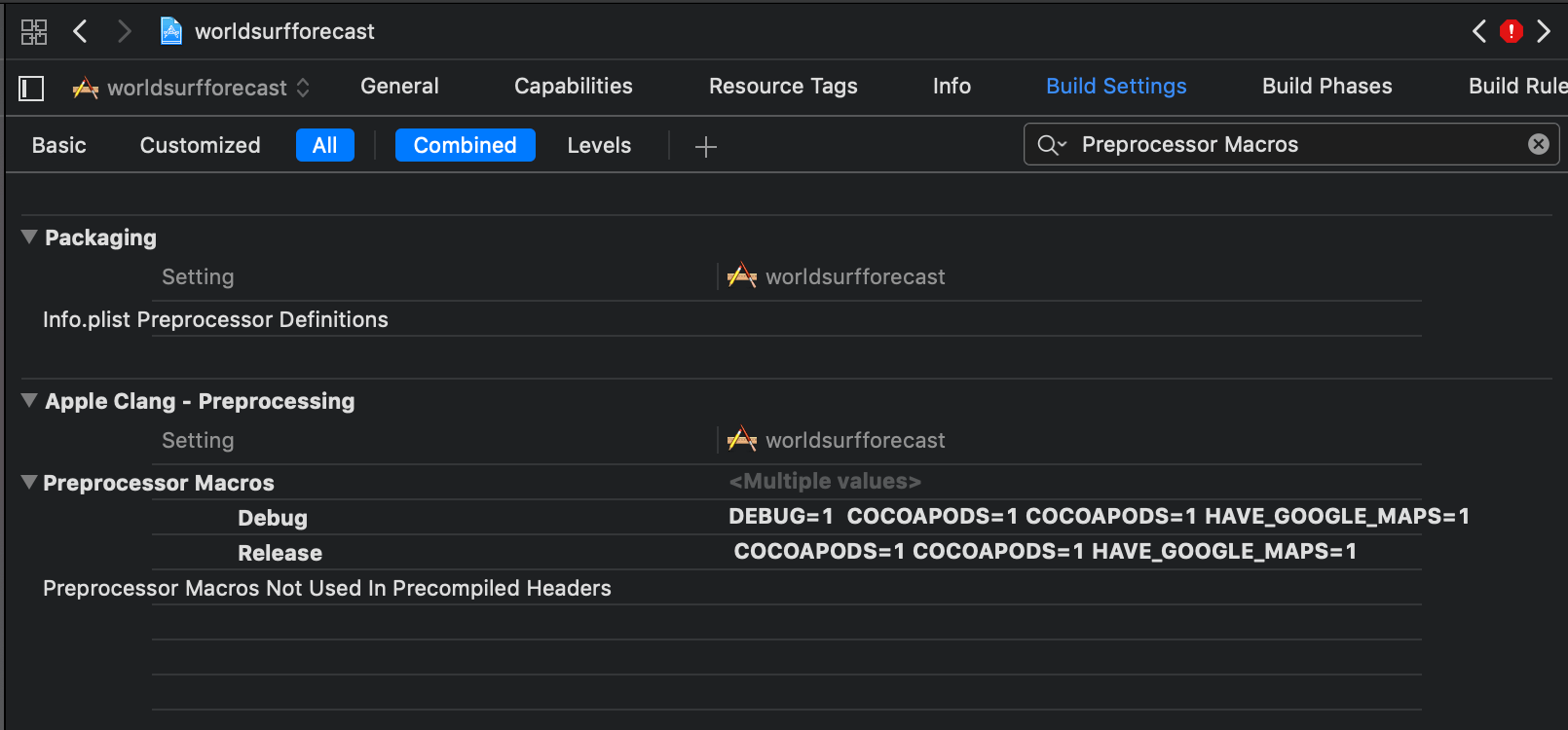
If you miss this step you'll get below error:
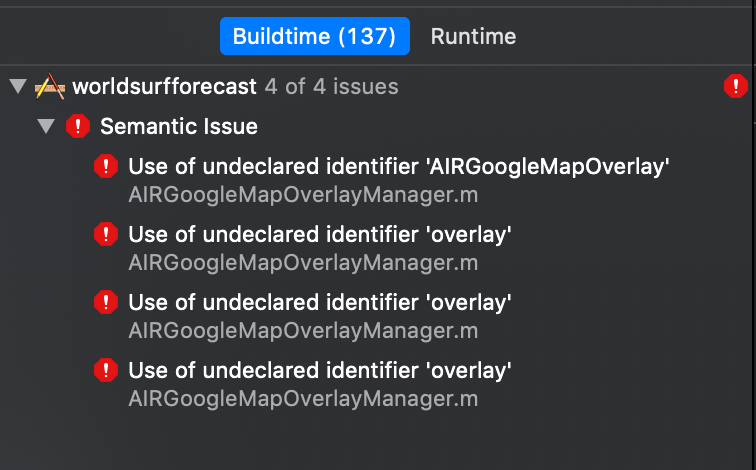
After this we're ready to change Apple maps into Google maps just by changing provider="google"
. If you remove this tag it'll switch back to Apple Maps.
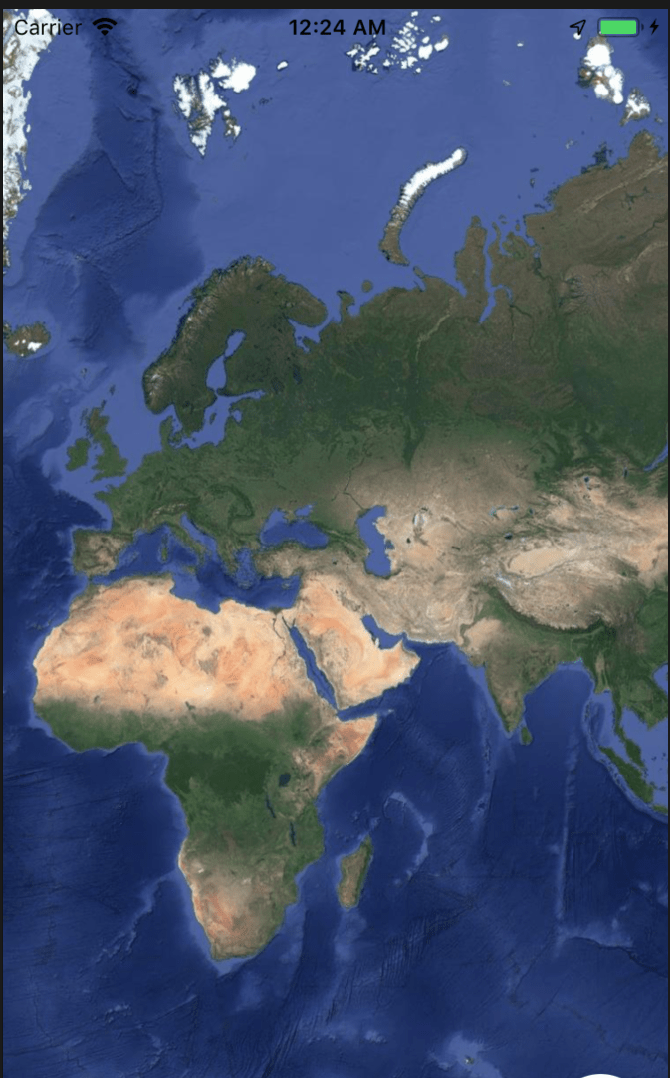
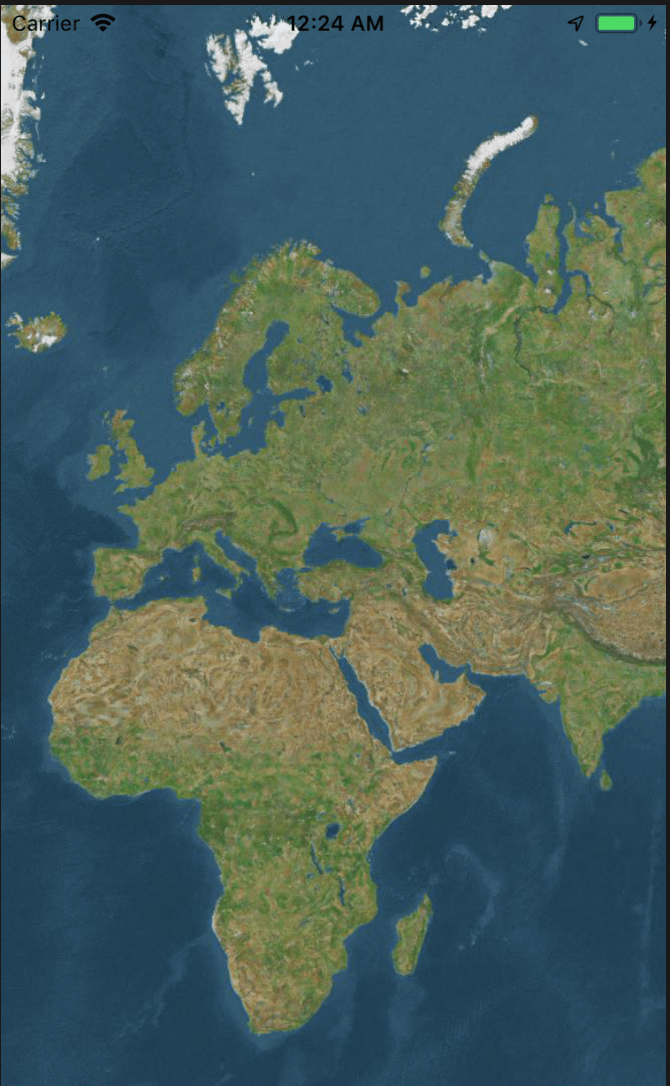