Python is great and it's not only for data scientists. You can also build desktop and mobile apps for Android, iOS, macOS, Linux and Windows. But it's very easy to get stuck because of dependencies and mismatching versions.
There are some tools that help us to make apps using Python.
CONDA
Conda is a dependency manager and makes it extremely easy to work with different versions of Python and its dependencies too. To be honest, at first I didn't like using it and did not find it very useful when I can manage everything using pip commands inside a docker container. Docker and Conda are of course completely 2 different things, but they both try to solve similar problems.
Docker is more general but still works quite good with Python dependency management especially when you're working with console or web applications. But, Conda is more specialised on managing Python and may be inevitable if you're working with graphical interfaces. So, with kivy library it's better to use Conda.
Usage is quite straightforward and does not have many commands to remember. So let's install Conda for our environment.
https://docs.anaconda.com/anaconda/install/
After installation, Conda will have a default environment called "base".
Commands | Example |
---|---|
Conda info, version etc. | conda info |
Create a new environment | conda create --name py35 python=3.5 |
List environments | conda env list |
Switch to an envirnment | conda activate kivy |
Remove an existing environment | conda env remove --name py35 |
Add a channel(respository) | conda config --add channels conda-forge |
Install a library | conda install cython |
Install a library specific version | conda install CUSTOMLIB=0.5.5 |
Install a library from a channel | conda install CUSTOMLIB -c conda-forge |
And now create a new environment for kivy and Python 3.5 using below command:
conda create --name kivy python=3.5
conda activate kivy
conda install kivy
If your terminal supports, you'll see the active environment. (similar to your active git branch). See below how my default environment (base) changed to kivy.
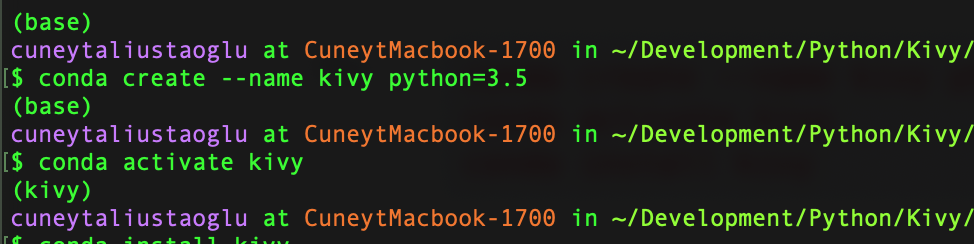
Kivy
Kivy technically is just another python library. But it's not an ordinary one. It's a framework for creating user interfaces that works on multiple platforms including Android, iOS, Windows, Linux and macOS. You can think it as an alternative to React Native, Flutter, Corona SDK etc. But Kivy is for Python lovers.
Installing Kivy with Conda is easy.
conda install kivy -c conda-forge
Let's create a very simple example from official documents. An accordion controller.
https://kivy.org/doc/stable/api-kivy.uix.accordion.html
Create a file called main.py
and fill it with below content:
from kivy.uix.accordion import Accordion, AccordionItem
from kivy.uix.label import Label
from kivy.app import App
class AccordionApp(App):
def build(self):
root = Accordion()
for x in range(5):
item = AccordionItem(title='Title %d' % x)
item.add_widget(Label(text='Very big content\n' * 10))
root.add_widget(item)
return root
if __name__ == '__main__':
AccordionApp().run()
And run:
python main.py
That's it. Just a few lines and we have a good looking UI that will work in any environment.
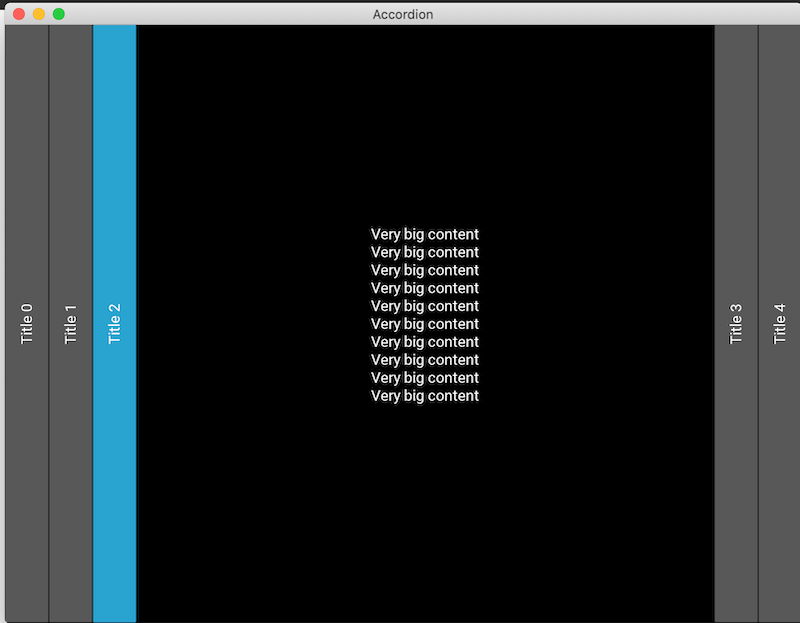
Now let's compile an iOS app from source code. Of course you will need macOS and Xcode for this and other than that we need a tool called kivy-ios.
git clone https://github.com/kivy/kivy-ios.git
cd kivy-ios
conda install --yes --file requirements.txt -c conda-forge
pip install pbxproj==2.5.1
# Build kivy-ios. This may take a while
python toolchain.py build python3 kivy
Note that toolchain.py file is inside this kivy-os folder. So, when you need to run it you may need to use either absolute or relative path unless you're already inside the folder. Also library pbxproj could not be found in any of the conda channels. So that's why I used pip. It's not good to mix pip and conda but if inevitable you can go for it. Last step when we build recipes may take a while.
Luckily we won't be building kivy-ios for every project. But we will use it to create the Xcode project what we'll be working on.
python toolchain.py create <title> <app_directory>
When you execute above command, make sure that app-folder is the one that contains the main.py file. I used it like below:
python /absolute/path/to/kivy-ios/toolchain.py create my-awesome-app /absolute/path/to/myproject
In your project folder next to main.py
, A directory named <title>-ios
is created. eg. my-awesome-app-ios. Find the xcodeproj and open. Click the run button and see the application is running in iOS simulator.
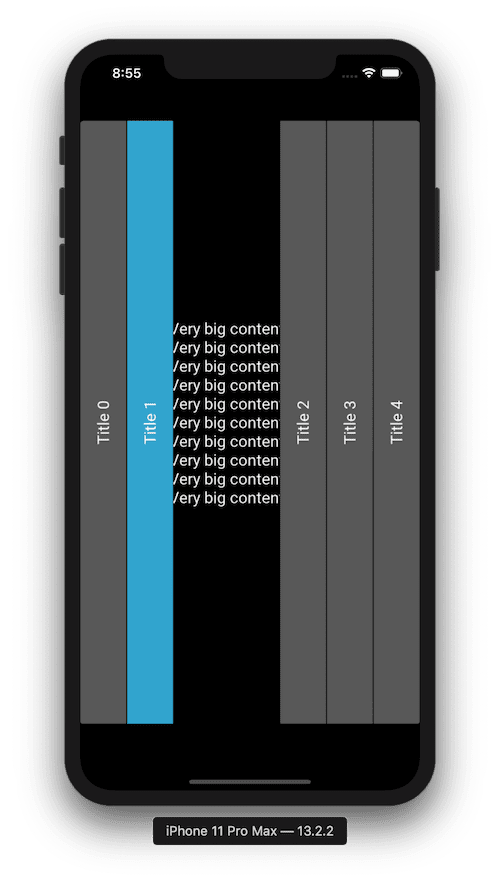
If the run button throws an error, try cleaning the project and running it again. That's what happened to me. Whenever you make a change to python file just click the run button again and it the changes will be reflected. And since this is an Xcode project, app signing and steps needed to submit an app to App Store is no different than a native app.
Note: I'm not an iOS fan that ignores Android completely but using buildozer for Android is quite troublesome and I couldn't manage to make it run on macOS. I'll do a Part-2 on creating deliverables for Android and other operating systems ;)